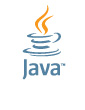
{filelink=5706}
/*
Java Programming for Engineers
Julio Sanchez
Maria P. Canton
ISBN: 0849308100
Publisher: CRC Press
*/
// File name: Area.java
//Reference: Chapter 5
//
//Java program to calculate the area of a circle
//Topics:
// 1. Using numeric variables and constants
// 2. Obtaining keyboard input
// 3. Displaying program data
// 4. Performing simple numeric calculations
//
//Requires:
// 1. Keyin class in the current directory
public class Area {
// Constant PI is defined at the class level
static final double PI = 3.141592653589793;
public static void main(String[] args) {
// Local variables
double radius, area;
// Input radius from keyboard
radius = Keyin.inDouble("Enter radius: ");
// Perform calculations and display result
area = PI * (radius * radius);
System.out.println("The area is: " + area);
}
}
//**********************************************************
//**********************************************************
//Program: Keyin
//Reference: Session 20
//Topics:
// 1. Using the read() method of the ImputStream class
// in the java.io package
// 2. Developing a class for performing basic console
// input of character and numeric types
//**********************************************************
//**********************************************************
class Keyin {
//*******************************
// support methods
//*******************************
//Method to display the user's prompt string
public static void printPrompt(String prompt) {
System.out.print(prompt + " ");
System.out.flush();
}
//Method to make sure no data is available in the
//input stream
public static void inputFlush() {
int dummy;
int bAvail;
try {
while ((System.in.available()) != 0)
dummy = System.in.read();
} catch (java.io.IOException e) {
System.out.println("Input error");
}
}
//********************************
// data input methods for
//string, int, char, and double
//********************************
public static String inString(String prompt) {
inputFlush();
printPrompt(prompt);
return inString();
}
public static String inString() {
int aChar;
String s = "";
boolean finished = false;
while (!finished) {
try {
aChar = System.in.read();
if (aChar < 0 || (char) aChar == '
')
finished = true;
else if ((char) aChar != '
')
s = s + (char) aChar; // Enter into string
}
catch (java.io.IOException e) {
System.out.println("Input error");
finished = true;
}
}
return s;
}
public static int inInt(String prompt) {
while (true) {
inputFlush();
printPrompt(prompt);
try {
return Integer.valueOf(inString().trim()).intValue();
}
catch (NumberFormatException e) {
System.out.println("Invalid input. Not an integer");
}
}
}
public static char inChar(String prompt) {
int aChar = 0;
inputFlush();
printPrompt(prompt);
try {
aChar = System.in.read();
}
catch (java.io.IOException e) {
System.out.println("Input error");
}
inputFlush();
return (char) aChar;
}
public static double inDouble(String prompt) {
while (true) {
inputFlush();
printPrompt(prompt);
try {
return Double.valueOf(inString().trim()).doubleValue();
}
catch (NumberFormatException e) {
System.out
.println("Invalid input. Not a floating point number");
}
}
}
}