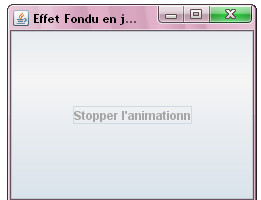
{filelink=10251}
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import javax.swing.Timer;
import java.awt.AlphaComposite;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import java.awt.BorderLayout;
public class FadeComponent extends JFrame
{
public FadeComponent()
{
JFrame f = new JFrame("Effet Fondu en java");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setSize(250, 200);
JPanel pnl=new JPanel();
pnl.add(new FadingButton("Cliquez ici pour me faire disparître")
, BorderLayout.SOUTH);
f.add(pnl);
f.setVisible(true);
}
public static void main(String argv[])
{
Runnable showMyGUI = new Runnable() {
public void run() {
new FadeComponent();
}
};
SwingUtilities.invokeLater(showMyGUI);
}
}
class FadingButton extends JButton implements ActionListener {
float alpha = 1.0f;
Timer timer;
int dureeAnimation = 5000;
long animationStartTime;
BufferedImage btnImage = null;
public FadingButton(String btnTexte) {
super(btnTexte);
setOpaque(false);
timer = new Timer(30, this);
addActionListener(this);
}
/*
* Cette méthode gère les cliques
* de lancement et d'arrêt de l'animation
*/
public void actionPerformed(ActionEvent ae) {
if (ae.getSource().equals(this)) {
// Démarrer l'animation
if (!timer.isRunning()) {
animationStartTime = System.nanoTime() / 1000000;
this.setText("Stopper l'animationn");
timer.start();
} else {
timer.stop();
this.setText("Démarrer l'animation");
alpha = 1.0f;
}
} else {
long currentTime = System.nanoTime() / 1000000;
long totalTime = currentTime - animationStartTime;
if (totalTime > dureeAnimation) {
animationStartTime = currentTime;
}
float fraction = (float) totalTime / dureeAnimation;
fraction = Math.min(1.0f, fraction);
alpha = Math.abs(1 - (2 * fraction));
repaint();
}
}
public void paint(Graphics g) {
if (btnImage == null || btnImage.getWidth() != getWidth()
|| btnImage.getHeight() != getHeight()) {
btnImage = getGraphicsConfiguration().createCompatibleImage(getWidth(), getHeight());
}
Graphics gButton = btnImage.getGraphics();
gButton.setClip(g.getClip());
super.paint(gButton);
Graphics2D g2d = (Graphics2D) g;
AlphaComposite newComposite = AlphaComposite.getInstance(AlphaComposite.SRC_OVER, alpha);
g2d.setComposite(newComposite);
g2d.drawImage(btnImage, 0, 0, null);
}
}