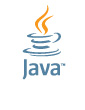
{filelink=10866}
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/****
* Le programme suspend et reprend
* un thread de manière non sécurisée
***/
public class ThreadEtat extends JFrame implements Runnable
{
// Les Objets a animer
private static final String[] listObjet =
{ "|", "/", "-", "\", "|", "/", "-", "\" };
private Thread monThread;
private JTextField mascotte;
public ThreadEtat()
{
mascotte = new JTextField();
mascotte.setEditable(false);
mascotte.setFont(new Font("Monospaced", Font.BOLD, 26));
mascotte.setHorizontalAlignment(JTextField.CENTER);
final JButton susprendre_anim = new JButton("Suspendre");
final JButton reprendre_anim = new JButton("Reprendre");
susprendre_anim.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
suspendre();
}
});
reprendre_anim.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
reprendre();
}
});
JPanel pnl = new JPanel();
pnl.setLayout(new GridLayout(0, 1, 3, 3));
pnl.add(mascotte);
pnl.add(susprendre_anim);
pnl.add(reprendre_anim);
setLayout(new FlowLayout(FlowLayout.CENTER));
add(pnl);
setTitle("Exemple de Thread");
setSize(320, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
// Méthode de suspension
private void suspendre()
{
if ( monThread != null )
{
monThread.suspend();
}
}
// Méthode de reprise
private void reprendre()
{
if ( monThread != null )
{
monThread.resume();
}
}
public void run() {
try {
monThread = Thread.currentThread();
int count = 0;
while ( true )
{
mascotte.setText(
listObjet[ count % listObjet.length ]);
Thread.sleep(200);
count++;
}
} catch ( InterruptedException x ) {
} finally {
monThread = null;
}
}
public static void main(String[] args)
{
ThreadEtat obj = new ThreadEtat();
Thread thr = new Thread(obj);
thr.start();
}
}