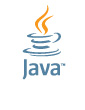
{filelink=4390}
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.channels.Channels;
import java.nio.channels.FileChannel;
import java.nio.channels.WritableByteChannel;
/**
* Copier le contenu d'un fichier vers un autre
* argument1: fichier source
* argument2: fichier de destination
*/
public class CopierFichier {
public static void main(String args[])
{
FileInputStream fin = null;
FileOutputStream fout = null;
try {
fin = new FileInputStream(args[0]);
FileChannel in = fin.getChannel();
WritableByteChannel out;
if (args.length > 1) {
fout = new FileOutputStream(args[1]);
out = fout.getChannel();
} else { // Aucun fichier de destination spécifié
out = Channels.newChannel(System.out);
}
// Obtenir la taille du fichier
long numbytes = in.size();
in.transferTo(0, numbytes, out); // Effectuer le transfert du fichier
} catch (IOException e) {
System.out.println(e);
} finally {
try {
if (fin != null)
fin.close();
if (fout != null)
fout.close();
} catch (IOException e) {
}
}
}
}