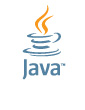
{filelink=10238}
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import javax.swing.Timer;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class AnimationBouton extends JFrame
{
public AnimationBouton()
{
JFrame f = new JFrame("Animation des composant en Java");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setSize(250, 200);
JPanel pnl = new JPanel();
pnl.add(new CustomButton("Lancer l'animation"));
f.add(pnl);
f.setVisible(true);
}
public static void main(String argv[])
{
Runnable showMyGUI = new Runnable() {
public void run() {
new AnimationBouton();
}
};
SwingUtilities.invokeLater(showMyGUI);
}
}
class CustomButton extends JButton implements ActionListener {
Timer timer;
int dureeAnimation = 5000; // Le bouton reste 5 secondes dans l'ombre
long animStartTime;
int translateY = 0;
static final int MAX_Y = 100;
/** Initialisation de JButton avec son texte */
public CustomButton(String jbuttonText)
{
super(jbuttonText);
setOpaque(false);
timer = new Timer(30, this);
addActionListener(this);
}
public void paint(Graphics g) {
g.translate(0, translateY);
super.paint(g);
}
/*
* Cette méthode gère les cliques
* de lancement et d'arrêt de l'animation
*/
public void actionPerformed(ActionEvent evt) {
if (evt.getSource().equals(this)) {
// Démarrer l'animation si le timer n'est pas encore d'exécution
if (!timer.isRunning()) {
animStartTime = System.nanoTime() / 1000000;
this.setText("Stopper l'animation");
timer.start();
} else {
timer.stop();
this.setText("Lancer l'animation");
translateY = 0;
}
} else {
long currentTime = System.nanoTime() / 1000000;
long totalTime = currentTime - animStartTime;
if (totalTime > dureeAnimation) {
animStartTime = currentTime;
}
float fraction = (float)totalTime / dureeAnimation;
fraction = Math.min(1.0f, fraction);
if (fraction < .5f) {
translateY = (int)(MAX_Y * (2 * fraction));
} else {
translateY = (int)(MAX_Y * (2 * (1 - fraction)));
}
repaint();
}
}
}