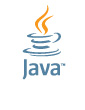
{filelink=10867}
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/****
* Le programme Stoppe un thread
* en cours d'exécution
***/
public class StopThread extends JFrame implements Runnable
{
// Les Objets a animer
private static final String[] listObjet =
{ "|", "/", "-", "\", "|", "/", "-", "\" };
private volatile boolean stopRequested;
private Thread monThread;
private JTextField mascotte;
public StopThread()
{
mascotte = new JTextField();
mascotte.setEditable(false);
mascotte.setFont(new Font("Monospaced", Font.BOLD, 26));
mascotte.setHorizontalAlignment(JTextField.CENTER);
final JButton stop_anim = new JButton("Stopper l'animation");
stop_anim.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
stopper();
}
});
JPanel pnl = new JPanel();
pnl.setLayout(new GridLayout(0, 1, 2, 2));
pnl.add(mascotte);
pnl.add(stop_anim);
setLayout(new FlowLayout(FlowLayout.CENTER));
add(pnl);
setTitle("Exemple de Thread");
setSize(320, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
// Arrêter le thread
private void stopper()
{
stopRequested = true;
if ( monThread != null )
{
monThread.interrupt();
}
}
public void run() {
try {
monThread = Thread.currentThread();
stopRequested = false;
int count = 0;
while ( !stopRequested )
{
mascotte.setText(
listObjet[ count % listObjet.length ]);
Thread.sleep(200);
count++;
}
} catch ( InterruptedException x )
{
Thread.currentThread().interrupt();
} finally {
monThread = null;
}
}
public static void main(String[] args)
{
StopThread obj = new StopThread();
Thread thr = new Thread(obj);
thr.start();
}
}