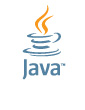
{filelink=1018}
import java.util.Date;
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Multipart;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
public class JavaMail_Mim
{
static String message1 = "Voici le contenu de msg1";
static String message2 = "voici le texte lié";
public static void main(String[] args)
{
Properties prop = new Properties();
prop.put("mail.smtp.host", "monserveumail");
prop.put("mail.from", "moi@example.com");
String to = "contact@exemple.com"; // destinataire
Session session = Session.getInstance(prop, null);
try {
// Créer un message
MimeMessage msg = new MimeMessage(session);
// Définir l'en tête du message
msg.setFrom();
msg.setRecipients(Message.RecipientType.TO, to);
msg.setSubject("Test d'envoi d'email multipart/mixed");
msg.setSentDate(new Date());
// Créer la partie contenu du premier message
MimeBodyPart mbp1 = new MimeBodyPart();
mbp1.setText(message1);
// Créer la partie contenu du second message
MimeBodyPart mbp2 = new MimeBodyPart();
mbp2.setText(message2);
// Créer un conteneur multipart pour les deux contenus
Multipart mp = new MimeMultipart();
mp.addBodyPart(mbp1);
mp.addBodyPart(mbp2);
// Ajouter le Multipart au message
msg.setContent(mp);
// Envoyer le message
Transport.send(msg);
} catch (MessagingException mex) {
mex.printStackTrace();
Exception ex = null;
if ((ex = mex.getNextException()) != null) {
ex.printStackTrace();
}
}
}
}